jQuery? You’ve got to toggle
Wednesday, June 17th, 2009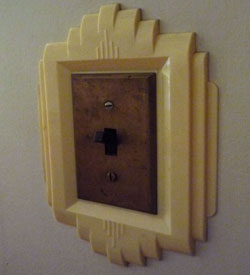
Toggling makes it easy to turn things on and off
Everyone likes writing jQuery for one main reason: it’s really easy to use. One feature that makes life a lot easier is toggling. Let’s say you have an image that you need to show when a button is clicked and then hide when it is clicked again. Instead of two separate functions, one to show and another to hide, use jQuery’s handy shorthand: toggle:
$(‘button’).click( function() {
$(this).toggle();
});
But jQuery’s support of toggling goes way beyond hiding and showing. It has toggleClass() which adds a class or removes it depending on if the class exists. Also slideToggle() which slides an object up and then down.
And besides these simple shorthands for existing functions, jQuery has a variety of support for more custom toggle functions. … (more…)