Controlling Animation Timing in jQuery
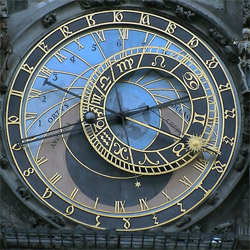
Photo credit: Simpologist
Animation and function timing can often seem like an uphill battle in Javascript. Thankfully jQuery’s variety of timing control mechanisms provide excellent alternatives to Javascript’s standard order of function processing.
With both callback functions and chainable methods, jQuery allows much greater control over animation timing than Javascript alone. Callback functions provide the ability to execute a function once an animation has completed, while chainable methods allow us to stack a series of operations on a single object. Combining the two, jQuery provides near perfect control over the timing of animations.
How to use callback functions
The first concept to understand about jQuery callbacks is that they are not actually a method like fadeOut()
but rather an argument: fadeOut( speed, callback )
. So if we wanted to fade out the body in 500 milliseconds, and then call the function myCallback()
we’d write:
$('BODY') . fadeOut( 500, myCallback );
Notice here that we did not add parentheses to myCallback
. This is because we are referencing the function myCallback()
, rather than calling it.
Alternatively, instead of passing a function name, we could declare a function on the fly using Javascript’s function(){}
syntax.
For example, if we’re making an image slideshow and want to fade out an image with id “image1” and afterwards fade in “image2”, we would write this callback:
$('#photo1') . fadeOut( 500, function() {
$('#photo2').fadeIn( 500 );
});
Take note of the syntax; we’re passing function(){some code here}
as our callback instead of a function name. Defining functions in this way is useful even in heavily object-oriented code, since passing a function as an argument prevents the ability to pass variables into the function.
Finally, we can use callbacks with all of the jQuery animation functions: animate()
, slideUp()
, fadeIn()
, etc. For more syntax info refer as always to the jQuery docs.
Why is the jQuery callback function important?
In normal Javascript, the processor calls functions serially, meaning one after another. For example:
firstFunc();
secondFunc();
This would call the function firstFunc()
followed immediately by secondFunc()
.
Javascript and therefore jQuery animations are almost always built using setInterval() and setTimeout(). For example, we might set an interval to move an image to the right 1 pixel every millisecond. If this were called within our function firstFunc()
, it would begin executing and then the Javascript engine would move immediately onto the next task: executing secondFunc()
.
Thankfully, jQuery’s callback function allows us to call a function after an animation has completed. This makes it one of the jQuery library’s most useful features.
jQuery’s chainable methods
In addition to callbacks, jQuery takes Javascript’s serial processing to the next level with chainable methods. This feature allows you to stack a series of functions on a single object. For example:
$('#image1') . fadeIn( 500 ) . addClass('active');
This would start to fade in the image, and then immediately add the class ‘active’, just as if you used Javascript’s normal serial processing:
$('#image1') . fadeIn( 500 );
$('#image2') . addClass('active');
However, the option of chainable methods makes the code more concise and readable, with the added bonus of limiting jQuery selector reuse.
Integrating callback functions and chainable methods
We certainly know a lot about jQuery animation timing now. Let’s apply all of this knowledge at once and integrate callbacks with chainable methods.
For practice, let’s apply an ‘animating’ CSS class to an image while executing an animation, and then remove the class when our animation is complete:
$('#image1') . animate( { 'width' : 0 }, function() {
$('#image1').removeClass( 'animating' );
}) . addClass('animating');
Pay careful attention to the order in which our jQuery functions get called:
1. First we call the animate()
function.
2. Once this initiates, we add the class ‘animating’.
3. Finally, when the animate()
completes, we remove the ‘animating’ class from the image.
jQuery’s various animation timing options have really allowed us to regulate when our functions and animations execute!
Tags: animation, callback, front end, interval, jQuery, processing, syntax, timing
May 9th, 2009 at 9:40 pm
Very helpful. Thank you!
May 13th, 2009 at 7:44 am
good article ! helped me to a great deal.
November 26th, 2009 at 2:54 am
Excellent!!! Very helpful…
Thank U…
December 14th, 2009 at 7:16 am
I’ve been looking everywhere for someone to explain it that simple, you fixed my long lasting problem. Thank you very very much.
April 6th, 2010 at 8:05 am
good explication about the jQuery callback on animations, really interesting. 😀