Supporting the Browser Back Button with Javascript
Tuesday, January 6th, 2009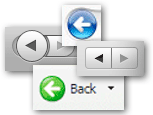
When developing a Javascript-heavy, AJAX-riddled site, I often run into a 2.0 type of problem: supporting the browser back button. While it’s wonderful to build a site where users are brought to various content pages without the window refreshing, this excellent user experience will be completely ruined if you hit the back button and it returns you to the root of the site (or worse the last site you visited).
Thus, integrating the browser becomes an integral part of any Javascript developer’s toolkit.
Making the browser recognize Javascript changes
The first problem we have to solve is making the browser respect a Javascript change as a page change. However we have to be sure not to change the actual location of the page, or our AJAX or Javascript experience will be shot.
One thing to look at is the window location’s … (more…)