jQuery? You’ve got to toggle
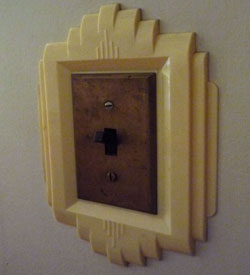
Toggling makes it easy to turn things on and off
Everyone likes writing jQuery for one main reason: it’s really easy to use. One feature that makes life a lot easier is toggling. Let’s say you have an image that you need to show when a button is clicked and then hide when it is clicked again. Instead of two separate functions, one to show and another to hide, use jQuery’s handy shorthand: toggle:
$('button').click( function() {
$(this).toggle();
});
But jQuery’s support of toggling goes way beyond hiding and showing. It has toggleClass() which adds a class or removes it depending on if the class exists. Also slideToggle() which slides an object up and then down.
And besides these simple shorthands for existing functions, jQuery has a variety of support for more custom toggle functions.
The toggle event
One handy application of toggling in jQuery is the toggle event. Use this to toggle between different functions when an element is clicked:
$('button').toggle(function() {
// function called on first click
}, function() {
// function called on second click
}, function() {
// function called on third click
});
This will toggle between calling these three functions as the ‘button’ element is clicked. Note that this can work with any number of functions, from 2 on up.
Using toggle within animate()
Another extremely useful toggling technique in jQuery is passing toggle as a CSS attribute in animate()
:
$('p').animate({
height: 'toggle'
});
This will create a default animation to toggle the height, which you may have noticed is the same as using the slideToggle()
function described above. But this method has its advantages: you have greater control, you can change any number of CSS attributes or perform any other task you need in your custom function in addition to toggling the height.
This shorthand works for a variety of CSS properties: opacity, height, width, top, bottom, left, right and pretty much anything else that you can toggle.
A practical application of toggling
Although slideToggle()
is a handy way to slideUp
and slideDown
, jQuery doesn’t provide a corresponding fadeToggle()
function. Fortunately we can write our own by passing ‘toggle’ as an attribute in the jQuery animate() method:
$('img').animate({
opacity: 'toggle'
});
This will toggle between fading this image in and out, depending on whether it has opacity.
Tags: animation, events, jQuery, shortcuts, technique, toggle
November 30th, 2009 at 2:39 am
Great resource! I learned a few things with this very well written article. Looking forward to more!